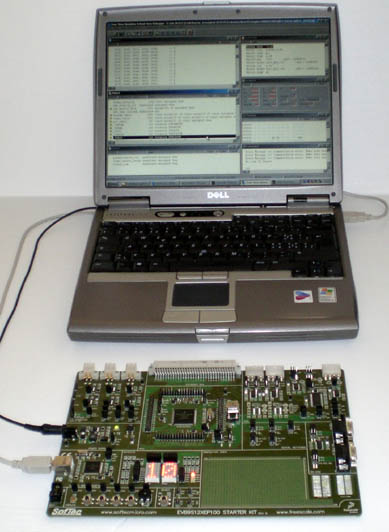
![]() |
|
Code examples |
High Level Code generation in C, C++, Visual C++ |
Boards with MC9S12XEP100 Demo Board connected to PC Evaluation-Board with MC9S12XEP100 Custom Board with MC9S12XEP100 1 PORTB blink or shift 1.0 PORTB blinking 1.1 PORTB shift left 1.2 PORTB shift left and right 2 Analog conversion 2.0 Analog conversion -> PORTA 2.1 Analog conversion - view steps 2.2 Send ADC-result to RS232 feedback:orgler@tin.it |
![]() a program to view the value of bytes over RS232 from HCS12 download scanbyte.zip #include <hidef.h> /* common defines and macros */ #include <MC9S12XEP100.h> /* derivative information */ #pragma LINK_INFO DERIVATIVE "mc9s12xep100" unsigned int iCounter; unsigned char cChan10; unsigned char cStatus; void main(void) { DDRB = 0xFF; // PORTB all outputs PORTB = 0xAA; // initialize PORTB DDRA = 0xFF; // PORTA all outputs DDRC = 0xFF; // only for EVBS12XEP100 DDRD = 0xFF; PORTC = 0; PORTD = 0; // SCI init SCI0BDH = 0; SCI0BDL = 13; // 9600 Baud at 4Mhz E-clock SCI0CR1 = 0; //Initialize for 8-bit Data Format SCI0CR2 = 0x0C; //[TIE,TCIE,RIE,ILIE,TE,RE,RWU,SBK] no interrupt // Configures the ATD peripheral ATD0CTL1 = 0x10; // 0001 0000 8 Bit Data Resolution and discharge before convert // 0000 1000 left justified data, 1 conversion sequence and non-FIFO mode ATD0CTL3 = 0x08; // fBUS=2MHz, fATDCLK = 1 MHz (PRESCALER=0) Select 24 Sample Time ATD0CTL4 = 0xE0; // 1110 0000 ATD0CTL5 = 0x0A; // start a conversion on channel 10 for(;;) { iCounter = 30000; // delay time while(iCounter > 0) iCounter--; PORTB ^= 0xFF; // PORTB blinking cChan10 = ATD0DR0H; PORTA = 0; if(cChan10 >= 0x10) PORTA=0x01; if(cChan10 >= 0x30) PORTA=0x03; if(cChan10 >= 0x50) PORTA=0x07; if(cChan10 >= 0x70) PORTA=0x0F; if(cChan10 >= 0x90) PORTA=0x1F; if(cChan10 >= 0xB0) PORTA=0x3F; if(cChan10 >= 0xD0) PORTA=0x7F; if(cChan10 >= 0xE0) PORTA=0xFF; ATD0CTL5 = 0x0A; // start a new conversion cStatus = SCI0SR1; SCI0DRL = cChan10; }// end loop } #include <hidef.h> /* common defines and macros */ #include <MC9S12XEP100.h> /* derivative information */ #pragma LINK_INFO DERIVATIVE "mc9s12xep100" unsigned int iCounter; unsigned char cChan10; void main(void) { DDRB = 0xFF; // PORTB all outputs PORTB = 0xAA; // initialize PORTB DDRA = 0xFF; // PORTA all outputs DDRC = 0xFF; // only for EVBS12XEP100 DDRD = 0xFF; PORTC = 0; PORTD = 0; // Configures the ATD peripheral ATD0CTL1 = 0x10; // 0001 0000 8 Bit Data Resolution and discharge before convert // 0000 1000 left justified data, 1 conversion sequence and non-FIFO mode ATD0CTL3 = 0x08; // fBUS=2MHz, fATDCLK = 1 MHz (PRESCALER=0) Select 24 Sample Time ATD0CTL4 = 0xE0; // 1110 0000 ATD0CTL5 = 0x0A; // start a conversion on channel 10 for(;;) { iCounter = 30000; // delay time while(iCounter > 0) iCounter--; PORTB ^= 0xFF; // PORTB blinking cChan10 = ATD0DR0H; PORTA = 0; if(cChan10 >= 0x10) PORTA=0x01; if(cChan10 >= 0x30) PORTA=0x03; if(cChan10 >= 0x50) PORTA=0x07; if(cChan10 >= 0x70) PORTA=0x0F; if(cChan10 >= 0x90) PORTA=0x1F; if(cChan10 >= 0xB0) PORTA=0x3F; if(cChan10 >= 0xD0) PORTA=0x7F; if(cChan10 >= 0xE0) PORTA=0xFF; ATD0CTL5 = 0x0A; // start a new conversion }// end loop } #include <hidef.h> /* common defines and macros */ #include <MC9S12XEP100.h> /* derivative information */ #pragma LINK_INFO DERIVATIVE "mc9s12xep100" unsigned int iCounter; void main(void) { DDRB = 0xFF; // PORTB all outputs PORTB = 0xAA; // initialize PORTB DDRA = 0xFF; // PORTA all outputs DDRC = 0xFF; // only for EVBS12XEP100 DDRD = 0xFF; PORTC = 0; PORTD = 0; // Configures the ATD peripheral ATD0CTL1 = 0x10; // 0001 0000 8 Bit Data Resolution and discharge before convert // 0000 1000 left justified data, 1 conversion sequence and non-FIFO mode ATD0CTL3 = 0x08; // fBUS=2MHz, fATDCLK = 1 MHz (PRESCALER=0) Select 24 Sample Time ATD0CTL4 = 0xE0; // 1110 0000 ATD0CTL5 = 0x0A; // start a conversion on channel 10 for(;;) { iCounter = 30000; // delay time while(iCounter > 0) iCounter--; PORTB ^= 0xFF; // PORTB blinking PORTA = ATD0DR0H; ATD0CTL5 = 0x0A; // start a conversion }// end loop } #include <hidef.h> /* common defines and macros */ #include <MC9S12XEP100.h> /* derivative information */ #pragma LINK_INFO DERIVATIVE "mc9s12xep100" unsigned int iCounter; int direction; void main(void) { DDRB = 0xFF; // PORTB all outputs PORTB = 0x01; // initialize PORTB direction = 1; for(;;) { iCounter = 60000; // delay time while(iCounter > 0) iCounter--; if(direction == 0) { PORTB = PORTB << 1; if(PORTB == 0 ) {direction = 1; PORTB= 0x80; } } else { PORTB = PORTB >> 1; if(PORTB == 0 ) {direction = 0; PORTB= 0x01; } } }// end loop } #include <hidef.h> /* common defines and macros */ #include <MC9S12XEP100.h> /* derivative information */ #pragma LINK_INFO DERIVATIVE "mc9s12xep100" unsigned int iCounter; void main(void) { DDRB = 0xFF; // PORTB all outputs PORTB = 0x01; // initialize PORTB for(;;) { iCounter = 60000; // delay time while(iCounter > 0) iCounter--; PORTB = PORTB << 1; // shift left if(PORTB == 0) PORTB = 0x01; }// end loop } #include <hidef.h> /* common defines and macros */ #include <MC9S12XEP100.h> /* derivative information */ #pragma LINK_INFO DERIVATIVE "mc9s12xep100" unsigned int iCounter; void main(void) { DDRB = 0xFF; // PORTB all outputs PORTB = 0x01; // initialize PORTB for(;;) { iCounter = 60000; // delay time while(iCounter > 0) iCounter--; PORTB ^= 0xFF; // invert all bits }// end loop } ![]() ![]() ![]() |